30 Days of JavaScript: Mastering Key Concepts Effectively
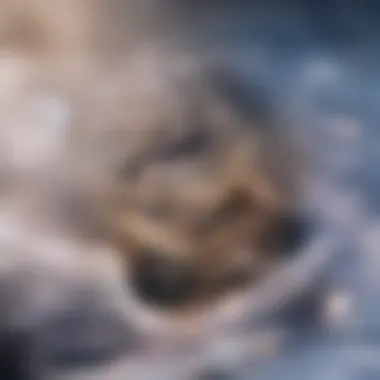
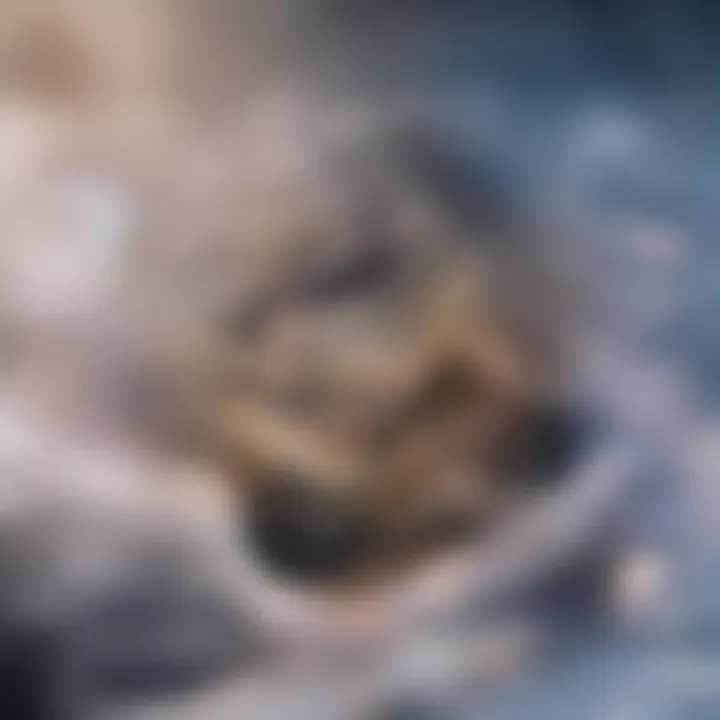
Intro
JavaScript has become a cornerstone of modern web development. Its versatility powers interactivity, enhances user experience, and forms the basis for numerous frameworks and libraries. This 30-day guide is designed to break down the complexities of JavaScript, making it accessible for both newcomers and those wishing to deepen their understanding.
The importance of mastering JavaScript in today’s technological landscape cannot be overstated. As websites evolve to require more dynamic features, a firm grasp of this language allows developers to create intricate user interfaces and engaging experiences. This structured approach will not only introduce essential concepts but also provide practical exercises and insights into application design.
Through consistent practice and application, learners will find themselves more capable in their ability to use JavaScript effectively. Each day's content builds upon the last, reinforcing knowledge while introducing new ideas. By the end of this period, participants will be equipped with the skills necessary to implement JavaScript in various real-world scenarios.
Key Focus Areas
- Fundamental principles of JavaScript
- Practical exercises that apply learned concepts
- Real-world scenarios illustrating JavaScript's role
- Strategies for solving common programming challenges
"JavaScript is not just a programming language but a key tool in web development that opens up new opportunities for creativity and innovation."
By the end of this journey, readers will not only recognize the core syntax and functions but also appreciate how these elements fit together to create powerful web applications.
Prelude to JavaScript
JavaScript is a cornerstone of modern web development. It enables interactivity, enhances user experience, and creates dynamic content. Understanding JavaScript is vital for anyone looking to succeed in the tech field today. In this guide, you will explore JavaScript's fundamental principles, which are crucial for both beginners and seasoned developers.
First, it is important to recognize the practical applications of JavaScript. Websites and applications leverage this language for functionalities such as form validation, animation, and even server-side programming. Mastering JavaScript can improve your ability to create engaging user interfaces and complex web applications.
Additionally, learning JavaScript opens doors to various frameworks and libraries. Knowledge of popular tools like React, Angular, and Vue can further enhance your coding skills. Each framework has its strengths, but they all rely on JavaScript as a base. Thus, a strong foundation in JavaScript is essential for utilizing these advanced tools effectively.
Through this article, readers will gain a detailed understanding of JavaScript's syntax, best practices, and methodologies. The structured approach offers both theoretical insights and practical exercises. This combination allows learners to engage with the language more effectively and apply knowledge in real-world scenarios.
History of JavaScript
JavaScript was created in 1995 by Brendan Eich while working at Netscape. Originally, it was designed to add interactivity to web pages. Over the years, it evolved significantly. The introduction of Ajax in the early 2000s marked a pivotal change, allowing for asynchronous data loading without refreshing the web page. This innovation paved the way for single-page applications.
In 2009, the evolution continued with the release of ECMAScript 5, which introduced robust features like JSON support and improved error handling. Later, ECMAScript 6 in 2015 brought significant enhancements — arrow functions, classes, and enhanced object properties. These changes have made JavaScript more powerful and versatile.
JavaScript's Place in Modern Development
In contemporary development, JavaScript holds a prestigious position. It is essential for building interactive websites and applications. Thanks to frameworks and libraries, JavaScript can be used not only for client-side development but also for server-side programming through Node.js.
Furthermore, JavaScript's ubiquity across all browsers makes it a preferred choice for developers. Unlike other programming languages, it does not require compilation, making it easier for developers to see the results of their code instantly. This immediacy fosters a rapid development cycle, which is critical in today’s fast-paced environment.
JavaScript remains the language of the web, effectively bridging frontend and backend development.
By consolidating your understanding of JavaScript, you position yourself to become an invaluable asset in any tech team. Mastering this language is a pathway to numerous opportunities and advancements in the world of software development.
Setting Up Your Environment
Setting up your environment is a crucial step when learning JavaScript. An optimized environment enhances productivity, minimizes errors, and provides the necessary tools to write, test, and debug code. This section will guide you through the essentials of creating a suitable setup that allows you to delve into JavaScript with confidence.
Choosing a Code Editor
The code editor is where you will spend much of your time writing and editing JavaScript code. Selecting the right editor can significantly affect your coding efficiency. Several popular options include Visual Studio Code, Sublime Text, and Atom. Each editor has unique features and capabilities that developers appreciate.
Visual Studio Code is widely regarded for its extensive support for plugins and robust community. It features IntelliSense for code completion, integrated terminal, and built-in Git support. Sublime Text is praised for its speed and clean interface, making for a smooth coding experience. Atom, GitHub's text editor, allows for collaborative coding.
When choosing a code editor, consider the following factors:
- Ease of use: It should be user-friendly and accessible for beginners.
- Customization: The ability to personalize settings and themes can enhance comfort while coding.
- Support for Extensions: A decent marketplace for plugins expands functionalities as needed.
Ultimately, your choice should align with your preferences and workflow needs. Taking time to explore various options would be beneficial.
Installing Node.js and npm
Node.js is an essential runtime for executing JavaScript outside the browser. It allows developers to run scripts server-side, which is particularly useful for building back-end services and tools. Along with Node.js comes npm, the Node Package Manager, which is critical for managing libraries and dependencies that your projects may require.
To install Node.js:
- Visit the official Node.js website at nodejs.org.
- Download the installer suitable for your operating system (Windows, macOS, or Linux).
- Follow the installation prompts to complete the setup.
- Verify the installation by running the command in your terminal. This should display the version of Node.js installed.
- Similarly, run to check that npm was installed correctly.
Both tools provide access to a vast ecosystem of libraries and frameworks that can simplify the development process. Mastery of Node.js and npm expands your capabilities as a JavaScript developer, allowing you to build modern applications effectively.
Setting up your development environment can drastically improve your coding experience and streamline the process of learning JavaScript.
Establishing a solid foundation in your environment prepares you for the journey ahead, where the focus can shift from the tools to the language itself. A well-set environment encourages experimentation and exploration of JavaScript principles.
Understanding Basic Syntax
The foundational aspect of programming in JavaScript is understanding its basic syntax. Mastering this allows for effective communication with the JavaScript engine and aids in the creation of functional web applications. Not only does grasping syntax pave the way for writing clean code, but it also enhances debugging capabilities and overall code quality. Without a solid grasp of syntax, developers face challenges in implementation and comprehension of more advanced concepts. Clear organization and familiarity with syntax lead to better collaboration and code maintenance.
Variables and Data Types
In JavaScript, a variable is a named storage for data values. Using variables, developers can store, modify, and retrieve data easily. Understanding the types of data that can be assigned to these variables is crucial. JavaScript supports several data types, including:
- String: Represents textual data, enclosed in single or double quotes.
- Number: Stands for both integers and floating-point numbers.
- Boolean: A logical data type that can hold only two values: true or false.
- Object: A complex data type that allows the grouping of values.
- Array: A special type of object used to store multiple values in a single variable.
- Undefined: Represents a variable that has been declared but not assigned a value.
Declaring variables in JavaScript can be done using , , or . Each keyword has its own significance and scope. For example, is used for variables that may change, while is used for immutable values. Here’s a simple declaration:
Mastering the use of variables and data types is important for constructing logic, managing data efficiently, and ultimately implementing functionality within applications.
Operators and Expressions
Operators in JavaScript perform operations on variables and values. They are categorized into several types:
- Arithmetic Operators: Used for mathematical calculations (e.g., +, -, *, /).
- Comparison Operators: Used to compare two values (e.g., ==, ===, >, ).
- Logical Operators: Used for logical operations (e.g., &&, ||, !).
- Assignment Operators: Used to assign values to variables (e.g., =, +=, -=).
Expressions are combinations of variables, values, and operators that are evaluated to produce a value. Understanding how to construct expressions with the appropriate operators is a core skill for developers. For instance:
By manipulating variables using these operators and expressions, developers can create complex logic for their applications. Consequently, a firm grasp of operators contributes significantly to writing efficient and effective JavaScript code.
"The simplicity in JavaScript syntax often belies the power and complexity of its applications."
Control Structures
Understanding control structures is crucial for any JavaScript developer. They dictate the flow of execution in programs. With control structures, you can say things like: "If this condition is true, do this; otherwise, do something else." They make your code dynamic, allowing for decision-making capabilities. You are not just executing step-by-step anymore; you are managing paths and actions based on data and conditions.
Conditional Statements
Conditional statements allow for branching logic in JavaScript. The most common conditional statements are the , , and . They enable the execution of blocks of code depending on whether a specified condition evaluates to true or false. For example:
This simple construct allows your application to react to different input values. Additionally, JavaScript also offers the statement, which can test multiple conditions more cleanly than a series of statements. It works well in cases where you have several potential outcomes based on the same variable.
"Conditional statements are foundational. They transform static code into responsive code that considers user input and behavior."
Loops in JavaScript
Loops are another fundamental control structure in JavaScript, enabling you to execute a block of code multiple times. The major types of loops in JavaScript include , , and . Each has its use case—whether you know the number of iterations in advance or need to continue looping until a condition is false.
For instance, a loop might look like this:
In this code, the loop runs five times, logging the index each time. A loop might be used when the exact number of iterations is not known ahead of time:
Control structures, particularly loops, are vital for tasks that involve iteration such as traversing arrays or collecting user input. They introduce the ability to cycle through data structures, making them integral to creating efficient and scalable applications. By mastering these concepts, you will significantly enhance your programming capability.
Functions and Scope
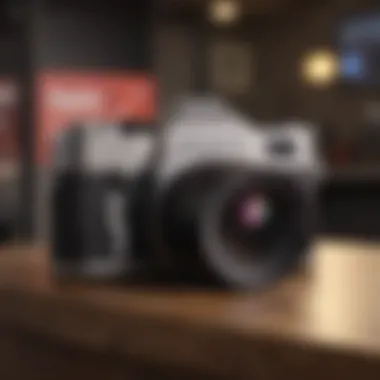
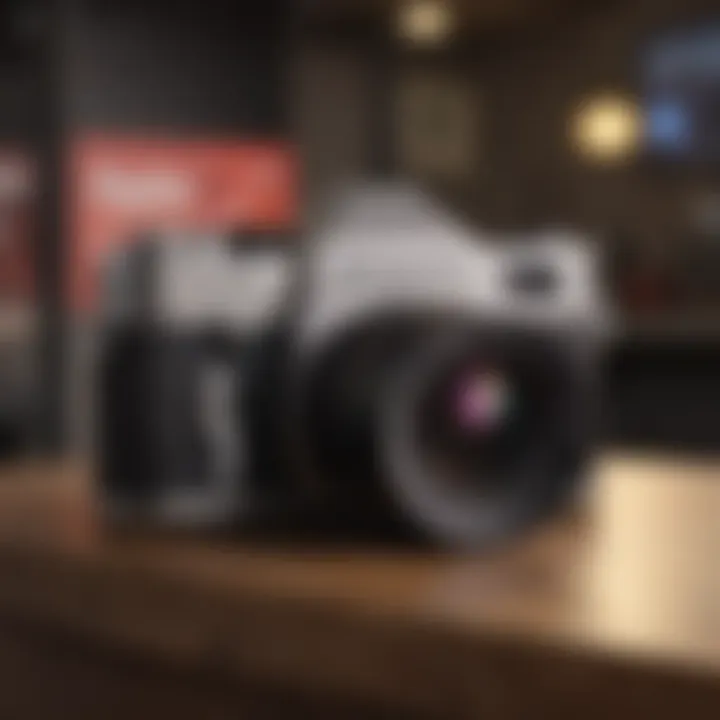
Functions and Scope play a crucial role in JavaScript. Understanding these concepts is essential for effective programming and ensures the development of efficient, manageable, and reusable code. Functions serve as the building blocks of JavaScript, allowing developers to encapsulate behavior and create modular code. Moreover, grasping scope helps in managing variable accessibility, which is vital in avoiding conflicts and maintaining clean code.
Function Declaration vs Expression
In JavaScript, a distinction exists between function declarations and function expressions.
A function declaration is a straightforward way to define a function. It includes the keyword , followed by a name and a list of parameters in parentheses. Here’s an example:
This method defines the function and hoists it, meaning it can be called before its definition in the code. On the other hand, a function expression involves assigning a function to a variable. This expression can be anonymous (without a name) or named. For example:
In this case, the function cannot be called until it is defined in the code. Using function expressions enhances flexibility. They can be passed as arguments and returned from other functions. Thus, both forms have their use cases, and knowing when to use each is important for a JavaScript developer.
Understanding Scope
Scope in JavaScript pertains to the visibility of variables in different parts of your code. There are three primary types of scope: global scope, local scope, and block scope.
Global scope means that a variable is accessible from any part of the code. This can lead to potential problems, particularly if two variables share the same name. Conversely, local scope is confined to a specific function. Variables defined inside a function are not accessible from the outside, which helps prevent naming conflicts.
Understanding these scope types helps manage variable lifetimes and access, making code more predictable. > "Proper scope management is vital for avoiding unintended consequences in your applications."
In summary, functions and scope are foundational to mastering JavaScript. By differentiating between function declarations and expressions, as well as grasping various scope levels, developers can write clearer, more efficient code.
Object-Oriented Programming
Object-Oriented Programming (OOP) is a significant paradigm in programming that focuses on the representation of objects. This approach to programming facilitates the organization of code in a way that mirrors real-world interactions and relationships. OOP enforces a modular structure, which can vastly improve code manageability and reuse. By understanding OOP principles in JavaScript, developers can leverage the language to build complex applications that are easier to manipulate, maintain, and enhance over time.
Creating Objects
In JavaScript, objects are fundamental building blocks of the language. They allow developers to group related data and functions together. There are several ways to create objects in JavaScript:
- Object Literal Syntax: This is the most straightforward way to create an object. You simply define key-value pairs enclosed in curly braces.
- Constructor Functions: Another method to create multiple objects of the same type involves using a constructor function. A constructor function is defined as a regular function but is designed to be called with the keyword.
- ES6 Classes: The class syntax introduced in ES6 provides a clearer and more elegant way to create objects and handle inheritance. Classes encapsulate data and functionality in a manageable unit.
These methods exemplify the flexibility JavaScript offers in creating objects. Mastering object creation is essential as it underpins every application built using the language.
Prototypes and Inheritance
Prototypes are a core feature of JavaScript that enables the implementation of inheritance. Each object has a prototype, which is another object from which it can inherit properties and methods. This allows for the creation of complex hierarchies and can significantly reduce redundancy in code.
- Prototype Chain: When accessing a property or method, JavaScript first looks at the object itself. If it cannot find it, the search continues up the prototype chain, looking through linked objects. This behavior allows for shared properties and methods across instances of an object.
- Constructor Prototypes: You can add methods to a constructor’s prototype, making them accessible to all instances of that constructor. This is an efficient way to ensure that all objects share methods without duplicating the same function every time a new instance is created.
- Inheritance with Prototypes: You can create a new constructor that inherits from an existing constructor. In this case, the child constructor can access properties and methods from the parent constructor. This is done via or by setting the prototype of one constructor to an instance of another.
Understanding prototypes and inheritance is crucial for any JavaScript developer looking to implement OOP concepts effectively. It provides a rich system for organizing code, allowing for extensions without modification of existing code.
Asynchronous Programming
Asynchronous programming is a fundamental concept in JavaScript that allows for efficient handling of operations that take time, such as fetching data from a server or processing large files. Understanding asynchronous programming is essential for developing responsive web applications. With users expecting quick interactions, implementing asynchronous behavior improves user experience and application performance. This section explores two major aspects of asynchronous programming: callbacks and promises.
Understanding Callbacks
A callback is a function that is passed as an argument to another function. This allows the second function to execute the callback at a designated point, usually after completing some operation. Callbacks enable JavaScript to perform tasks without blocking the main execution thread.
For example, consider a scenario where you need to load data from an API:
In this code, simulates a data-fetching operation with and calls the function once the operation is complete. This approach is simple but can lead to issues like callback hell, where multiple nested callbacks make the code hard to read and maintain.
Promises and Async/Await
Promises provide a cleaner alternative to callbacks. A promise is an object that represents the eventual completion or failure of an asynchronous operation. A promise can be in three states: pending, fulfilled, or rejected. Using promises helps avoid the messy nested structures associated with callbacks.
Here’s an example of how to create and use a promise:
In this snippet, is a promise. The method handles fulfillment, while manages rejections. This structure keeps the code readable and manageable.
Using , syntactic sugar built on promises, provides an even clearer way to write asynchronous code. \nTo utilize it, simply declare a function with the keyword and use in front of promise calls:
With , the code resembles synchronous code, making it easier to read and reason about. This way, developers can handle asynchronous operations reliably, improving the quality of web applications.
Understanding asynchronous programming is crucial as it fundamentally alters how we interact with web APIs and improve user experiences.
With these insights, we lay a solid foundation for mastering JavaScript's asynchronous capabilities, enhancing how we write and organize our code.
Working with the DOM
Working with the Document Object Model (DOM) is a crucial aspect when learning JavaScript. The DOM represents the structure of a web page as a tree of objects. Each object corresponds to a part of the document, such as elements, attributes, and text. Understanding how to manipulate the DOM allows developers to create dynamic and interactive web experiences.
Selecting and Manipulating Elements
Selecting and manipulating elements in the DOM is fundamental in web development. JavaScript provides several methods to select DOM elements, enabling developers to alter the content, style, and attributes of these elements easily.
- Common Selection Methods:
- : Used to select a single element by its ID.
- : Selects elements with a specified class name, returning a collection.
- : Provides a way to select the first element matching a CSS selector.
- : Returns all elements that match a specified CSS selector.
Once elements are selected, developers can manipulate them using various properties and methods. For example:
- Changing Text Content:
- Changing Styles:
- Adding / Removing Classes:
Manipulating the DOM is essential for creating responsive user interfaces. However, it is worth noting that excessive or inefficient DOM manipulation can lead to performance issues.
Event Handling
Event handling is another vital area when working with the DOM. JavaScript allows developers to interact with user actions like clicks, key presses, or form submissions. By responding to these events, developers can enhance user experience significantly.
- Adding Event Listeners:
Developers use the method to attach an event handler to a specific event. Here’s how it works: - Types of Events:
- Click events
- Key events (keydown, keyup)
- Mouse events (mouseover, mouseout)
- Form events (submit, input)
Handling events allows developers to control the workflow of applications, making it possible to create interactive features. It is essential to understand event propagation and how to manage it properly to ensure efficient event handling.
Understanding DOM manipulation and event handling is key to building interactive and functional web applications.
In summary, working with the DOM represents a significant part of mastering JavaScript. Selecting and manipulating elements, along with effective event handling, lays the groundwork for creating dynamic web applications.
Error Handling
Error handling is a significant aspect of programming in JavaScript. It allows developers to anticipate problems that may arise during execution and to gracefully manage these issues. A well-implemented error handling strategy can significantly enhance the user experience by preventing abrupt application crashes and providing informative feedback to users. Furthermore, it also aids in the debugging process, enabling developers to identify and rectify issues with greater ease.
Understanding the types of errors that can occur is crucial. There are generally three categories of errors: syntax errors, runtime errors, and logical errors. Syntax errors happen when your code does not adhere to the proper structure, which generally prevents any execution. Runtime errors occur while the program is running, often leading to unexpected crashes or results. Logical errors, on the other hand, do not stop execution but result in incorrect outcomes. Each of these error types requires different strategies for handling effectively.
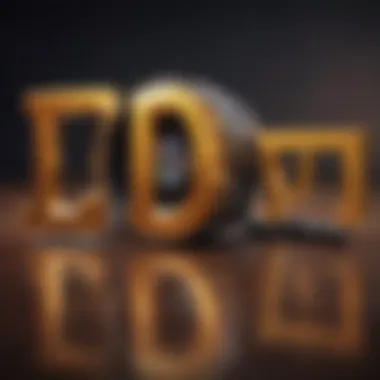
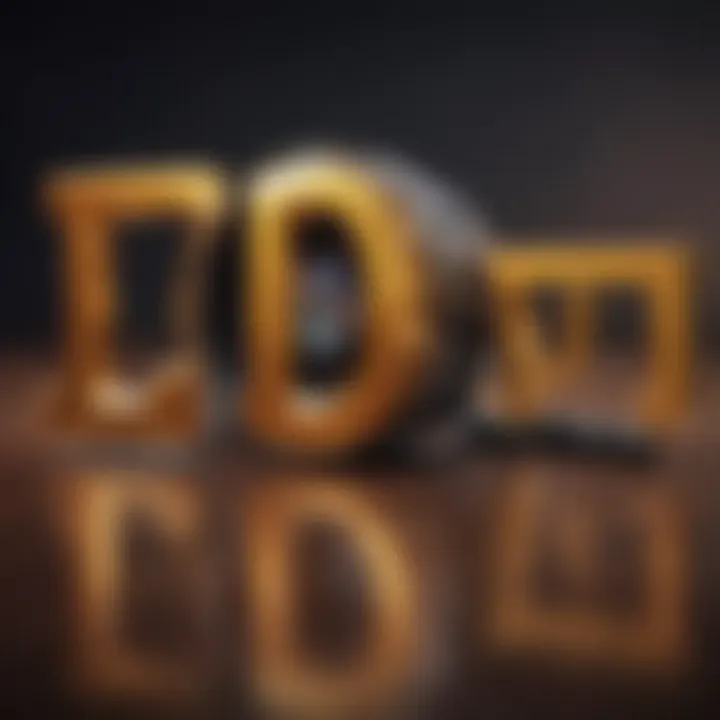
Types of Errors
Syntax Errors
These errors occur during the parsing of code and are usually flagged by the JavaScript engine before the program is run. Common syntax errors include missing brackets, misspelled keywords, or incorrect punctuation. JavaScript’s syntax checker identifies these issues, allowing developers to fix them before execution.
Example of a syntax error:
In the above code, the use of instead of creates a syntax error, as this line should perform a comparison.
Runtime Errors
Runtime errors are more problematic as they occur during program execution. These errors can stem from issues like dividing by zero or attempting to access invalid elements within an array. When a runtime error happens, it usually raises an exception that interrupts the flow of the program. Developers must anticipate these scenarios to manage them effectively.
Example of a runtime error:
Here, trying to access a property of an variable leads to a runtime error.
Logical Errors
Logical errors can be the most difficult to trace. These errors occur when the program runs without crashing, yet produces incorrect results. They often stem from a misunderstanding of actual requirements versus the implemented logic. Careful debugging and testing are necessary to identify these types of errors.
Example of a logical error:
This function is intended to add two numbers but has been written to subtract them instead.
Using trycatch
The statement is a fundamental mechanism for handling errors. This structure allows developers to execute a block of code inside the part where errors might occur. If an error is thrown, control is passed to the block, where developers can define how to handle that error. This encapsulation creates a controlled environment where unexpected behaviors can be managed without crashing the program.
Example of trycatch
In this example, if encounters an error during execution, it is caught in the block. Here, the developer outputs a meaningful error message to the console instead of allowing the application to fail silently or crash.
Implementing error handling not only protects your applications but also makes them more robust. By using the structure, developers can ensure that users receive appropriate feedback, allowing them to rectify issues quickly while maintaining engagement with the application.
JavaScript in the Browser
JavaScript's role in the browser is pivotal for creating dynamic and interactive web applications. As a core technology alongside HTML and CSS, JavaScript enhances the user experience by allowing web pages to respond to user actions in real time. This section explores the browser environment in which JavaScript operates and introduces debugging tools crucial for developing effective code.
Understanding the Browser Environment
When we talk about the browser environment, we refer to the context in which JavaScript code runs. Every web browser provides a JavaScript engine that interprets and executes the code. Browsers like Chrome, Firefox, and Safari utilize engines such as V8, SpiderMonkey, and JavaScriptCore respectively. Understanding this environment is essential for developers.
The Document Object Model (DOM) is a key component; it represents the structure of a web page. JavaScript can manipulate the DOM, adding elements, changing styles, or responding to user interactions. The ability to interact with HTML elements dynamically transforms static pages into responsive interfaces, enhancing usability and engagement.
In addition to the DOM, the browser's window object provides several critical functionalities. It includes methods for managing the browser, such as , , and timers like and . Knowing how to leverage these features will improve functionality significantly.
Using Console and Debugging Tools
Debugging is an integral part of the development process. Modern browsers come equipped with powerful developer tools. These tools include a console for logging outputs, debugging JavaScript, and monitoring performance issues. Using the console, developers can view messages, execute JavaScript snippets, and troubleshoot errors.
The Elements panel allows you to inspect and modify the DOM structure and CSS styles live. This feature lets you see changes immediately, which is invaluable for debugging layout issues. The Network tab assists in monitoring API calls and resource loading times, providing a clearer picture of the performance of your web applications.
Key Debugging Steps:
- Open Developer Tools (usually F12 or right-click and select Inspect)
- Use the Console to log messages and track variables
- Breakpoints can be set in the Sources panel to pause execution and examine the call stack
"Effective debugging is not just about fixing errors; it's about understanding why they occurred in the first place."
In summary, mastering the browser environment and utilizing debugging tools will empower developers to write more efficient and effective JavaScript code. It fortifies their ability to diagnose issues, leading to faster development cycles and less frustration.
Frameworks and Libraries
Frameworks and libraries are crucial in the JavaScript ecosystem. They enhance development efficiency and power the building of complex applications. Using them allows developers to avoid reinventing the wheel by leveraging existing solutions. They can streamline processes such as DOM manipulation, state management, and routing.
When developers use frameworks and libraries, they benefit from a solid foundation. This foundation offers pre-built functions and components that work seamlessly together. It can save significant time and keep the codebase clean and organized.
Additionally, these tools often come with robust community support. Issues can be resolved quickly through forums or documentation. This makes it easier for developers, especially those in the early stages of their careers, to learn and troubleshoot problems. Here are some key considerations when working with frameworks and libraries:
- Learning Curve: Each framework or library has specific patterns and idiosyncrasies that may require learning.
- Performance: Some libraries might add unnecessary weight to an application. It's important to choose wisely based on project needs.
- Long-Term Support: Verify that a library or framework is actively maintained. This ensures future compatibility and access to the latest features.
"Using a framework does not eliminate the need to understand JavaScript. It complements your knowledge and enhances your productivity."
Prologue to Common Libraries
JavaScript libraries serve as a toolset, offering reusable code for common tasks. Among the most notable libraries are jQuery and Lodash. jQuery simplifies DOM manipulations and events, making it more intuitive for developers. Lodash provides utility functions that make dealing with arrays and objects easier and more efficient. These libraries sometimes bridge the gap for developers who prefer not to dive deep into vanilla JavaScript.
- jQuery: Known for its simplicity in handling events and Ajax requests, jQuery significantly reduces code complexity.
- Lodash: Focused on performance optimization, Lodash allows developers to handle data structures with efficiency.
These libraries can greatly enhance productivity and let developers focus more on application Features rather than common utilities.
Exploring Frameworks like React and Vue
React and Vue are two frameworks that have gained immense popularity in recent years. They both facilitate building user interfaces but approach the task differently.
React, developed by Facebook, follows a component-based architecture. This means that developers build encapsulated components that manage their state. React emphasizes the concept of the virtual DOM. Changes in the UI are efficient due to this method. This leads to better performance, especially in applications with dynamic content.
Vue, by contrast, offers a more approachable framework. Its ease of integration into existing projects is one of its biggest advantages. With a gentle learning curve, Vue provides reactive data binding and a component-based system similar to React's architecture. Both frameworks have strong communities, which means that resources, plugins, and support are abundant.
Considerations for choosing between React and Vue include:
- Community Size: React has a larger community and more resources available.
- Ease of Adoption: Vue can be easier for newcomers due to its simpler syntax and structure.
- Project Requirements: Understand the specific needs; both frameworks can meet various project goals effectively.
In summary, frameworks and libraries play a pivotal role in modern JavaScript development. Familiarizing oneself with them can lead to better coding practices and more robust applications.
Storing Data and APIs
Storing data and accessing APIs are critical components in web development. Understanding these concepts allows developers to create applications that can handle user data effectively and interact with other services over the internet. As applications become more complex, the need to manage, retrieve, and store data efficiently arises. Moreover, the ability to access APIs extends the functionality of applications and provides a seamless experience for users.
Working with JSON
JavaScript Object Notation, or JSON, is a lightweight format used for data interchange. JSON is easy to read and write, and it is widely adopted across the web. When working with APIs, data is typically exchanged in JSON format. Understanding how to parse and stringify JSON is fundamental.
- Parsing JSON helps in transforming a JSON string into a JavaScript object. This process allows manipulation and access to properties.
- Stringifying a JavaScript object converts it into a JSON string, making it suitable for storage or sending over a network.
Here is simple code snippet that demonstrates parsing and stringifying JSON in JavaScript:
Utilizing JSON properly enhances communication between your web applications and external APIs. Developers should be proficient in using JSON since it is integral for data management in modern web applications.
Accessing APIs
APIs (Application Programming Interfaces) allow different software systems to communicate with each other. They are essential for enabling functionalities like retrieving weather data, user profiles, or even transaction histories. For JavaScript developers, mastering how to access APIs is crucial.
- To access an API, you typically use the function in JavaScript. This function returns a promise that resolves to the response of the request.
- Handling the response properly often involves checking the status code and converting the response to JSON.
Here's an example of using the API:
Through this approach, the developer can dynamically pull in data from various sources, enriching the user experience. It is vital to consider factors such as error handling, rate limiting, and authentication, particularly when dealing with third-party APIs. API access broadens the possibilities of what can be achieved with JavaScript, merging data in meaningful ways.
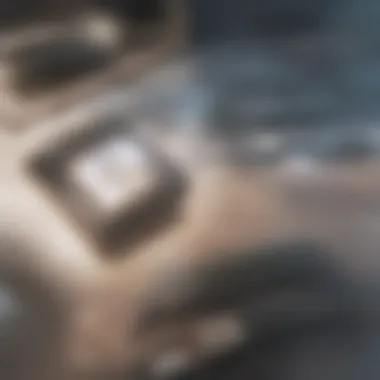
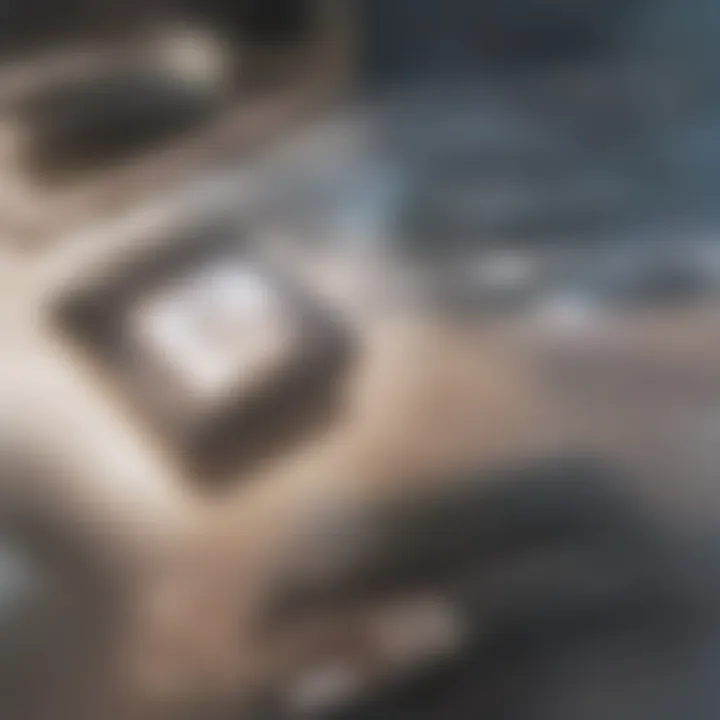
Version Control with Git
Version control is a critical aspect of any programming project. Git is the most popular version control system used today. Its importance lies in the ability to track changes, collaborate with others, and manage project versions efficiently. For developers learning JavaScript, understanding Git can significantly enhance their workflow and project management skills.
Git keeps a history of all changes made to the codebase. This allows developers to revert to previous versions when needed, which can save a lot of time and effort in debugging. Additionally, it helps in maintaining a record of contributions from multiple developers, making collaboration seamless.
When working on projects, issues can arise from working on the same files simultaneously. Git addresses this concern by enabling branching and merging, providing a way to work on features in isolation before integrating them into the main codebase. This modular approach is especially beneficial for larger projects, where different developers can work on different features without interfering with each other's code.
Foreword to Git
Git was created by Linus Torvalds in 2005. A decentralized version control system, it allows multiple developers to work on a project without unnecessary interference. The concept of "snapshots" is fundamental to Git. Instead of saving versions of files separately, Git saves information about changes made to the file. This approach reduces file size and improves efficiency.
Install Git from the official website. Once installed, you can verify the installation by running the command in your terminal. Basic configuration is also needed to set your user name and email:
Common Git Commands
Understanding common Git commands is essential for effective use. Here are some fundamental commands:
- : Initializes a new Git repository in your project directory.
- : Creates a copy of an existing repository.
- : Stages changes in the specified file for the next commit.
- : Saves staged changes in the repository with a descriptive message.
- : Displays the current state of the repository. You can see which changes are staged, unstaged, or untracked.
- : Uploads committed changes to a remote repository.
- : Fetches and merges changes from a remote repository into your local branch.
Using these commands effectively can provide a solid foundation for managing your projects. Learning Git may take time, but it is worth the effort and will prove to be an invaluable skill in your development journey.
Optimization Techniques
Optimization techniques are crucial in JavaScript development. They enhance application performance, improve user experience, and ensure efficient resource utilization. In web development, speed matters. Users expect fast, responsive applications. Sluggish performance can lead to user frustration and increased bounce rates. Thus, understanding and implementing optimization techniques is indispensable for modern developers.
Performance Considerations
When optimizing JavaScript, performance considerations are the first step. Key factors include:
- Load time: The time it takes for a webpage to fully load.
- Execution time: How fast scripts run in the browser once loaded.
- User experience: How users perceive the smoothness and responsiveness of the application.
To address these factors, developers can employ various strategies. First, analyzing bottlenecks using browser developer tools is essential. This step provides insights into script execution time and rendering performance.
Profiling helps identify slow functions, allowing developers to focus on optimizing these areas. Additionally, it's essential to minimize DOM interactions, as these can significantly impact performance. Reducing the number of changes made directly to the DOM will often lead to better performance outcomes.
Minification and Bundling
Minification and bundling are fundamental techniques in optimizing JavaScript code.
- Minification: This process involves removing all unnecessary characters from the source code without changing its functionality. Spaces, line breaks, and comments are stripped away. The resulting file is much smaller, which generally leads to faster load times.
For instance, consider the following example:
After minification, it becomes:
- Bundling: This technique combines multiple JavaScript files into a single file. This reduces the number of HTTP requests the browser needs to make. Fewer requests lead to quicker load times. Bundled files also tend to load in order, which can help with dependencies.
Both minification and bundling are essential for optimizing web applications. They not only reduce load time but also enhance the overall user experience. Implementing these techniques can give developers a significant edge in creating efficient, high-performing applications.
"Optimizing your code is about improving performance without compromising functionality."
Testing Your Code
Testing code is a crucial phase in software development. It ensures that the written code performs as expected, enhancing reliability, preventing bugs, and improving overall quality. In the context of JavaScript, which is widely utilized for web development, testing becomes even more essential. The dynamic nature of JavaScript allows for rapid changes, but that also means there is a higher chance for introducing errors. Therefore, implementing robust testing techniques is vital.
Effective testing can save time and resources in the long run. With a well-structured testing framework, developers can identify issues early in the development process. This helps in maintaining code quality and facilitates easier maintenance. Moreover, reliable tests allow for more confident iterative development, as modifications can be made with assurance that previously established functionality remains intact.
The following subsections will introduce you to various testing frameworks and methodologies, helping you to write effective tests for your JavaScript applications.
Foreword to Testing Frameworks
Testing frameworks provide a structured environment for developers to write and execute tests. JavaScript has several popular frameworks such as Jest, Mocha, and Jasmine. Each framework has its own strengths and use cases.
Jest is a popular choice for testing React applications but is versatile enough to be used with any JavaScript project. It comes with a built-in assertion library and supports features like snapshots for testing UI components.
Mocha is flexible and can be customized to fit a variety of testing styles. It allows tests to be organized in a straightforward manner, making it user-friendly.
Jasmine offers a behavior-driven development approach. It doesn't require a DOM and can be used to test JavaScript code in any environment.
When selecting a framework, consider factors such as the project type, complexity, and individual preferences. A good understanding of these frameworks will aid in establishing a solid foundation for effective testing methodology.
Writing Effective Tests
Writing effective tests involves more than just executing code; it's about ensuring accuracy, maintainability, and clarity. Effective tests should verify that the code behaves as expected under various scenarios. Here are some key considerations for writing effective tests:
- Clarity: Name tests clearly to reflect their purpose. This makes it easier for others to understand the context and function of each test.
- Isolation: Tests should run independently of each other. This helps in identifying faults more easily and prevents a single failing test from affecting others.
- Coverage: Strive for comprehensive test coverage. While achieving 100% coverage may be unrealistic, aim for a high percentage to cover various parts of the codebase.
- Use Assertions: Assertions serve as checkpoints to validate conditions. Employing a rich set of assertions helps in clearly defining expected outcomes.
- Frequent Testing: Integrate testing into the development workflow. Running tests frequently helps in catching errors early and fosters a habit of maintaining quality.
Writing tests can initially seem burdensome. However, the long-term benefits of having a reliable codebase outweigh these initial challenges. Establishing a routine for testing within your workflow will contribute significantly to the resilience of your JavaScript applications.
"Testing is not just a phase but a continuous process that ensures software quality throughout its lifecycle."
By systematically employing these principles and utilizing frameworks, developers can create reliable tests that enhance the effectiveness of their JavaScript projects.
Building Projects
Building projects represents a pivotal stage in learning JavaScript. It allows learners to transition from understanding theoretical concepts to implementing them in practical scenarios. This process is crucial because it not only solidifies understanding but also fosters creativity and innovation. When coding, students encounter real-world problems that require them to think critically and develop solutions that align with project goals.
In engaging with projects, one must consider several specific elements. First, the choice of project influences the scope of learning. The project should incorporate a variety of concepts learned over the previous days, such as functions, error handling, asynchronous programming, and interaction with the DOM. Moreover, projects provide a platform to experiment with frameworks and libraries, further broadening a developer’s skill set.
The benefits of building projects include:
- Practical Application: Students see how their coding skills translate into functional applications.
- Portfolio Development: Well-structured projects act as tangible evidence of skills for potential employers.
- Enhanced Problem Solving: Real-world challenges inevitably arise, allowing individuals to strengthen their analytical skills.
Apart from these benefits, it’s also essential to acknowledge key considerations when selecting a project. One must assess the complexity of the project relative to their current skill level. Overly ambitious projects may lead to frustration, while simplistic ones might not engage sufficient depth of understanding. Aim for projects that push boundaries, yet remain within a reachable scope for continual learning.
"Learning JavaScript through projects cultivates an environment of continuous growth and exploration, which is fundamental to mastery."
Choosing Your Project
When approaching project selection, it is vital to align with personal interests and goals. Projects that are personally motivated not only make the development process enjoyable but often lead to higher quality outcomes. Start by brainstorming topics that resonate, be it games, productivity tools, or web applications.
Consider these factors while choosing your project:
- Purpose: What is the goal of your project? Define whether it’s for learning, portfolio purposes, or personal use.
- Scope: Evaluate the time you are willing to invest. A simple JavaScript game can yield many learning opportunities without excessive complexity.
- Community Support: Look for projects that have a supportive community or resources available for guidance. This will be essential when facing obstacles.
Applying What You've Learned
Once your project is underway, the next goal is to implement the knowledge effectively. Start by breaking the project down into manageable segments. This helps in systematically applying the principles learned throughout your JavaScript journey. Incorporate best practices such as maintaining clean code, commenting, and adhering to a clear structure.
Within this stage, incorporate different elements learned from the previous sections of the article, like error handling mechanisms using , or using promises. Document your process; this will help in understanding how solutions evolve and aid in future projects.
In summary, building projects propels practical understanding of JavaScript. Choose relevant projects thoughtfully, applying learned concepts to ensure depth of knowledge and skillfulness. Engage with shared resources and communities for better support throughout the development process.
Resources for Continued Learning
Continued learning is critical for JavaScript developers at all stages, from beginners to experienced professionals. Resources available can shape the learning journey significantly. Not only do they provide fundamental knowledge, but they also offer updated practices and concepts as the industry evolves. By engaging with various resources, learners can deepen their understanding of JavaScript and its applications in modern web development.
Books and Online Courses
Books and online courses are traditional yet invaluable learning tools. They offer structured content ranging from foundational principles to advanced topics. When selecting books, consider titles that outline current JavaScript best practices, as the language can change rapidly. For instance, "Eloquent JavaScript" by Marijn Haverbeke is a respected text that guides readers through JavaScript with clarity and depth. Additionally, books like "JavaScript: The Good Parts" by Douglas Crockford focus on the most effective features of the language.
Online courses act as an excellent hands-on complementary resource. Platforms like Coursera, Udemy, and Codecademy provide interactive coding exercises, quizzes, and projects. By engaging in practical scenarios, learners can consolidate their knowledge effectively. Look for courses that cover assessments, as they can help track progress over time.
Online Communities and Forums
Participating in online communities and forums provides learners with insights beyond standard literature. These spaces allow developers to exchange ideas, troubleshoot problems, and share experiences. Websites like Reddit have dedicated subreddits for JavaScript where users can post questions and receive timely advice from fellow learners and experts alike.
Moreover, forums such as Stack Overflow have extensive question-and-answer sections focused on specific programming challenges. Engagement in these communities encourages problem-solving skills and fosters collaborations that can lead to deeper insights into JavaScript.
"The beauty of contributing to forums comes from the shared knowledge that can enhance both your problem-solving skills and understanding of JavaScript."
Overall, combining books, online courses, and community involvement creates an enriching environment for honing JavaScript skills. Leveraging diverse resources ensures comprehensive coverage of topics and fosters continuous growth in the field.